Java SE 11 Developer Certification (1Z0-819) Training
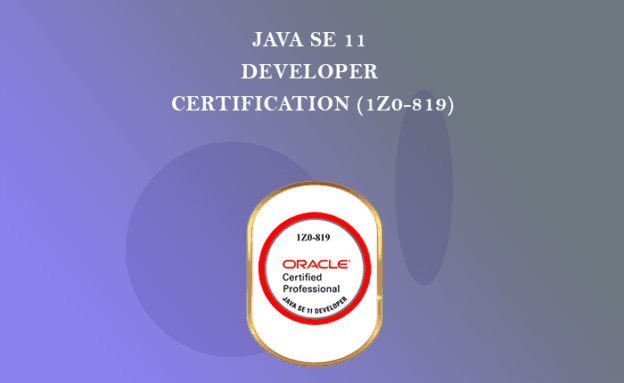
About Course
The Java SE 11 Developer Certification (1Z0-819) validates proficiency in Java programming skills, covering core Java SE 11 concepts such as variables, operators, and control structures, along with object-oriented programming, exceptions, and I/O operations. Aimed at Java developers, it demonstrates expertise in application development, enhancing career opportunities in Java-based roles across various industries by ensuring mastery of fundamental Java programming skills and best practices. Certification holders are recognized for their ability to design, develop, and maintain Java applications efficiently, contributing to robust and scalable software solutions that meet business needs effectively.
What Will You Learn?
- 1. In-Demand Skills
- 2. Career Advancement
- 3. Efficient CRM Management
- 4. Data Security
- 5. Workflow Automation
- 6. Reporting Insights
- 7. Job OpportunitiesHours On Demanded Videos
Course Content
Creating a Simple JAVA Program
-
Introduction
-
Course Info
-
Why Java 11?
-
Java Certification – Which Courses do I need?
-
Oracle Exam Codes – What you need to Know
-
Which Vendors JDK 11 Should you Use?
-
Which IDE should you Use?
-
Packages and Imports
-
Fully Qualified Class Name, Single Type Import and Type Import on Demand
-
Fully Qualified Class Name, Static Type Import and Static Import on Demand
-
Imports and Packages: Out of the Ordinary Concepts
-
Static Imports and Packages: Out of the Ordinary Concepts
Understanding JAVA Technology and the Environment
-
Understanding Java Technology and the Environment
Working with JAVA Primitive Data Types and String API’S
-
Section Introduction
-
Primitive Data Type Recap
-
Declaring Primitive Types
-
Local Variable Initialization
-
Narrowing and Widening
-
Casting
-
Declare and Initialize Variables: Out of the Ordinary Concepts
-
Quiz – Variable Initialization
-
Quiz – Variable declaration and initialization
-
Scope
-
More on Scope
-
Local Variable Scope: Out of the Ordinary Concepts
-
Quiz – Scope of local variables
-
Local Variable Type Inference
-
Local Variable Type Inference: Out of the Ordinary Concepts
-
Quiz – Local variable type inference
-
String Recap
-
Creating Strings
-
String Concatenation
-
Manipulating Strings
-
Text Search in String
-
Other String Manipulation
-
Replacement Methods and Text Transformation
-
Creating and Manipulating Strings: Out of the Ordinary Concepts
-
Quiz – String equality using “=” and .equals.
-
Quiz – String variables
-
Manipulate Data Using the StringBuilder
-
StringBuilder: Out of the Ordinary Concepts
-
Quiz – StringBuilder creation and concatenation, as well as concat methods
-
Quiz – StringBuilder constructors and the getChars() method
Using Operators and Decision Constructs
-
Section Intro
-
Java Operator Overview
-
Pre and Postfix Increment and Decrement Operators
-
Binary Operators Overview
-
Binary Operators Code Part 1
-
Binary Operators Code Part 2
-
Java Operators: Out of the Ordinary Concepts
-
Quiz – implications of operator results and type promotion
-
Quiz – pre and post-fix increment/decrement operators
-
if else Decision Construct
-
switch Decision Construct
-
Java Control Statements: Out of the Ordinary Concepts
-
Quiz – if statement constructions
-
Quiz – the switch statement
-
Loop Structures
-
for Loop: Out of the Ordinary Concepts
-
Quiz – the for loop statement
-
Quiz – the for loop statement
Working with JAVA Arrays
-
Introduction to Working with Java Arrays
-
Array Declaration and Initialization Overview
-
Array Declaration and Initialization
-
Manipulating Arrays
-
Array Search Methods
-
Manipulating Data in Arrays
-
Array Data Transformation Methods
-
Two Dimensional Arrays
-
Arrays: Out of the Ordinary Concepts – Unboxing
-
Arrays: Out of the Ordinary Concepts – Lists
-
Arrays: Out of the Ordinary Concepts – Summary of Copying
-
Quiz – array declaration, initialization, and indexing
-
Quiz – two dimensional arrays and static methods on the Arrays class
Describing and Using Objects and Classes
-
Section Introduction
-
Declare and Instantiate
-
Coding Classes and Garbage Collection
-
Quiz – dereferencing of objects and the eligibility of objects to be garbage
-
Quiz – declaring reference variables
-
Defining the Structure of a Class
-
More Declaration Examples and Initializer Blocks
-
Initializers and Static Initializers in Code
-
Class Structures: Out of the Ordinary Concepts
-
Quiz – constructors, initializers and static initializers
-
Quiz – class declarations, and static members of a class
-
Read or Write to Object Fields
-
Read or Write to Object Fields Code Examples
-
Quiz – static fields and static initializers
-
Quiz – static fields, their initialization, and accessing them
Creating and Using Methods
-
Section Introduction
-
Methods, Constructors, Arguments and Return Types
-
Methods Pass by Value and Constructors
-
Methods: Out of the Ordinary Concepts
-
Quiz – constructors
-
Overloaded Methods
-
Determining which Overloaded Methods gets called
-
Overloaded Methods: Out of the Ordinary Concepts
-
Quiz – overloaded method and constructors
-
Quiz – overloaded methods
-
Static Keyword on Methods and Fields
-
Detailed Static Keyword Example
-
Quiz – static keyword applied to methods and fields
-
Quiz – static keyword in association to the final modifier
Applying Encapsulation
-
Section Introduction
-
Applying Access Modifiers
-
Access Modifiers in Code
-
Access Modifiers: Out of the Ordinary Concepts
-
Quiz – knowledge of modifiers on the class level
-
Apply Encapsulation Principles to a Class
-
Apply Encapsulation Principles: Out of the Ordinary Concepts
-
Quiz – access modifiers on methods and the implications to classes
-
Quiz – definition of a java bean
Reusing Implementations through Inheritance
-
Section Introduction
-
Subclasses and Superclasses
-
Creating and Using Subclasses and Superclasses
-
Subclasses and Superclasses: Out of the Ordinary Concepts
-
Quiz – Accessing a simple method on a subclass
-
Quiz – different access modifiers on the subclass
-
Create and Extend Abstract Classes
-
Abstract Classes: Out of the Ordinary Concepts
-
Quiz – extending an abstract class
-
Quiz – Implementing abstract methods
-
Polymorphism
-
Polymorphism Code
-
Polymorphism: Out of the Ordinary Concepts
-
Quiz – Overriding methods
-
Polymorphism Casting Object vs Reference
-
Polymorphism Casting Mistakes and Upcasting
-
Polymorphism: Out of the Ordinary Casting
-
Polymorphism: Out of the Ordinary Generics
-
Quiz – Casting both primitives and reference variables
-
Quiz – Casting in calls to methods
Programming Abstractly through Interfaces
-
Section Introduction
-
Creating and Implementing Interfaces
-
Interfaces: Out of the Ordinary Concepts
-
Extending Interfaces: Out of the Ordinary Concepts
-
Quiz – Interfaces within Java
-
Quiz – Structure of the interface
-
Distinguish Class and Interface Inheritance
-
Code Examples Distinguish Class and Interface Inheritance
-
Quiz – Class inheritance from interface including abstract classes
-
Declare and Use List and ArrayList Instances
-
ArrayList Methods and Data Manipulation
-
ArrayList toArray Method
-
ArrayList toArray and Other Methods
-
List and ArrayList Exam Gotchas
-
Quiz – ArrayList declarations
-
Quiz – List.copyOf method
-
Anonymous Classes
-
Lambda Expressions
-
Coding Lambda Expressions
-
Multiple Parameters for Lambda Expressions
-
java.util.function Interfaces and Lambda Expressions
-
Quiz – The definition of a functional interface
-
Quiz – Simple lambda expression
Handling Expectations
-
Section Introduction
-
Exception Handling Overview
-
Exception Handling in Code
-
Try-catch Blocks and Program Flow
-
Try Finally
-
Create and Invoke Methods that Throw Exceptions
-
Exceptions: Out of the Ordinary Concepts
-
Exceptions Finally Clause and Summary
-
Exceptions in Static and Instance Initializers
-
Quiz – Local variable initialization in try/catch/finally declarations
-
Quiz – Checked and unchecked exceptions
Understanding Modules
-
Section Introduction
-
Describing the Modular Java Development Kit
-
Examining Modules from the Command Line
-
Creating, Compiling and Running Modules
-
Command Line Overview and Multiple Modules
-
Enabling Access Between Modules
-
Module Info Exports
-
Module Info Exports Qualified
-
Module Graphs
-
Quiz – Module relationships
-
Quiz – Module options with the various tools
Extro Info- Installation Videos and Source Code
-
Installing JDK 11, IntelliJ IDEA, and Command Line
-
Completed Code for all Programs
Bonus
-
Bonus Lecture and Information
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
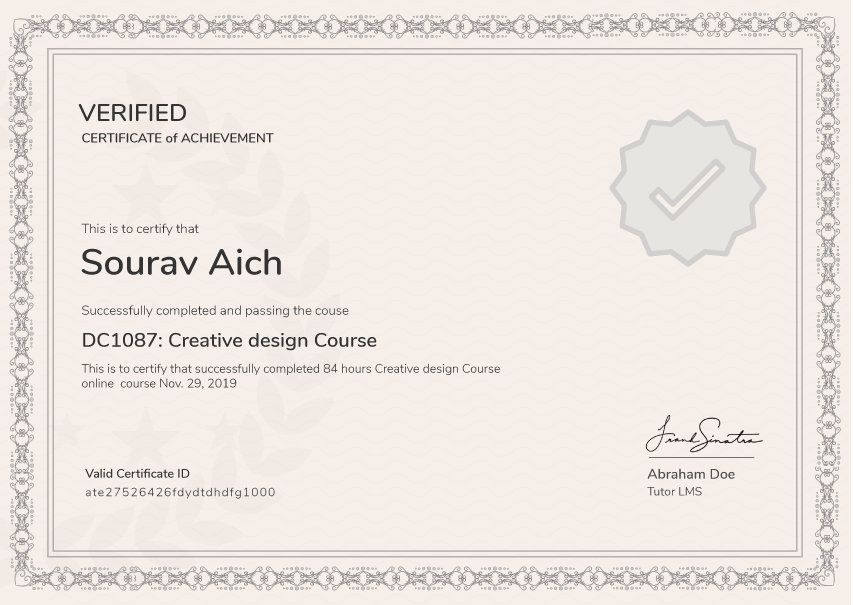